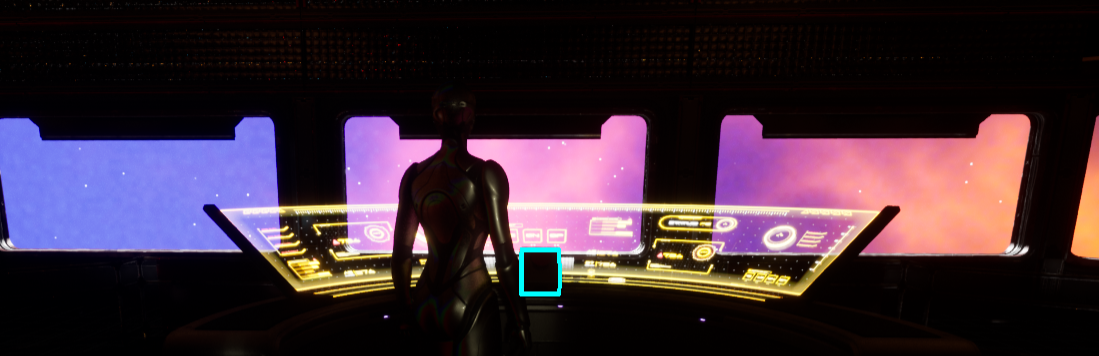
Space Station
A downloadable game
Space Station
This demo was created by Angelo Davis
The component source code can be found on my GitHub.
This is my first project while on the Mastered boot camp.
This project aims to show off some basic gameplay functionality coded using a combination of blueprints and C++ in Unreal Engine 5.
Controls: WASD for movement. F for interact. Mouse to look around.
Assets credit
Project Highlights
Character
The character has movement, look and interact controls bound using C++. Blueprints have been used to bind the interact and hover interface messages to the corresponding events.
AMyCharacterCPP::AMyCharacterCPP()
{
// Set this character to call Tick() every frame. You can turn this off to improve performance if you don't need it.
PrimaryActorTick.bCanEverTick = false;
//creating a collision sphere
CollisionSphere = CreateDefaultSubobject<uspherecomponent>(TEXT("CollisionSphere"));
CollisionSphere->SetupAttachment(GetMesh()); //connect collision sphere to character mesh
}
GetClosestInteractable function:
AActor* AMyCharacterCPP::GetClosestInteractable()
//Gets closest actor with the tag "Interactable" overlapping with the collision sphere.
{
AActor* ClosestActor = nullptr; //initiating closest actor
float ClosestDistance = 100000000; //large value so that the function works even if there is just one actor
TArray<aactor*> OverlappingActors; //initiating overlapping actor list
CollisionSphere->GetOverlappingActors(OverlappingActors);
for (AActor* Actor : OverlappingActors) {
//Get the distance between the character mesh and the actor
float ThisDistance = FVector::Dist(Actor->GetActorLocation(), GetMesh()->GetComponentLocation());
if (ThisDistance < ClosestDistance && Actor->ActorHasTag(TEXT("Interactable"))) {
ClosestDistance = ThisDistance; //overwrite distance if a subsequent distance is smaller
ClosestActor = Actor; //overwrite actor pointer if subsequent actor is closer
}
}
return ClosestActor;
}
This function returns the closest interactable actor overlapping with a collision sphere connected to the character code. This was written to return the closest interactable actor each tick, meaning the player can intuitively interact with the closest interactable actor to the player. This solves any problems that could otherwise arise if you had two or more interactable actors overlapping with the collision sphere on the player.
Interactable interface
The implementation of the interactable interface allows the player to interact with a variety of actors that can do different things all bound to the same "Interact" and "Hover" events. For example, the doors in this demo open, but interacting with the button triggers a dispatch that opens the blinds.
Interactable Door
The door C++ class contains the open / close functionality which has been exposed to blueprint. The child blueprint maps the interface to the open/close functionality and the hover functionality which highlights the door and shows the description widget.
Door tick function
void ADoor::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
//Opening and closing the door
if (ShouldMove) {
FVector CurrentLocation = ADoor::GetActorLocation();
ShouldClose = false; //Whether the door should automatically close after being opened
FVector NewLocation = FMath::VInterpConstantTo(CurrentLocation, TargetLocation, DeltaTime, Speed);
ADoor::SetActorLocation(NewLocation);
if (CurrentLocation == TargetLocation) {
SetShouldMove(false);
if (CurrentLocation == EndLocation)
{
ShouldClose = true;
//starting timer for the door to automatically close if left open
if (!GetWorld()->GetTimerManager().IsTimerActive(TimerHandle)) {
GetWorld()->GetTimerManager().SetTimer(TimerHandle, CloseTimer, CloseDelay, false);
}
}
}
}
}
The door tick function handles linearly interpolating between the current and target location. The interpolation speed and delay before automatically closing have been exposed to blueprints for configuration in the editor.
The target location is defined in terms of of a move offset relative to the door, meaning that the actor will always open in the same local direction, regardless of world rotation or translation.
Interactable Button
When the interact event is triggered on the button, this calls a dispatch to open all the blinds.
Calling Dispatch
Binding event to dispatch
Status | Prototype |
Author | Angelo Davis |
Leave a comment
Log in with itch.io to leave a comment.